Senior .NET Software Engineer
Indie Game Developer
Over 20 years experience creating business solutions for the government and the private sector


Blazor Server 403 Forbidden Errors
Tuesday, July 4, 2023
PROBLEM
Kept receiving 403 forbidden errors when moving code to production.
I believe the issue was related to copying the .NET 7 code into a location where .NET 6 code lived. I tried the code below which was UNSUCCESSFUL.

Blazor Server Timeout/Reconnect Issues
Tuesday, July 4, 2023
PROBLEM
When moving the code into production I started receiving connection issues.
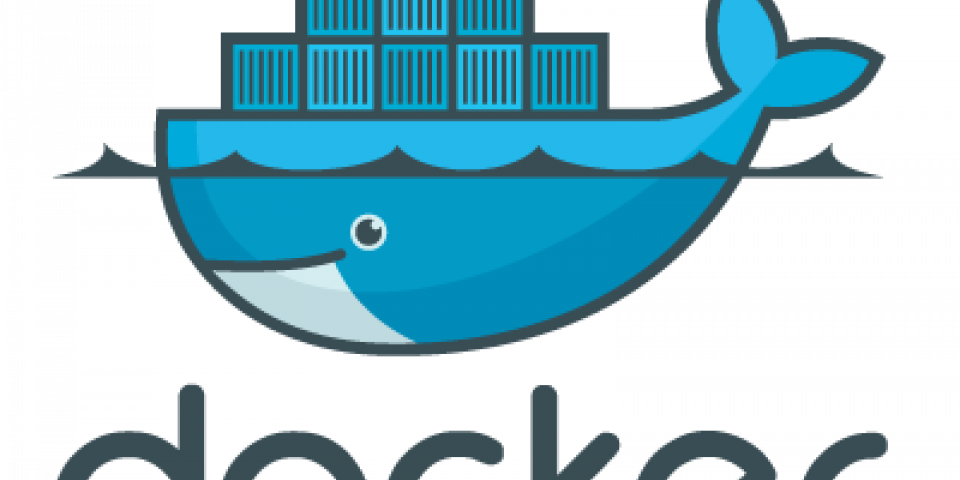
Docker Install and website setup
Saturday, July 18, 2020
Environment
- Ubuntu Linux 18.04
- Docker version 19.03
- ASP.NET Core 3.1
- Azure
Docker Installs
Windows Desktop Installation
Docker Desktop for Windows can be downloaded from Docker Hub
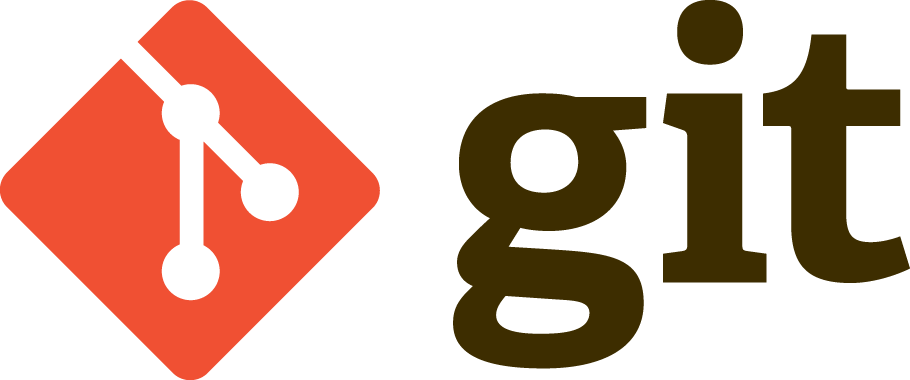
Git - .gitignore issues
Wednesday, December 11, 2019
A common issue you may have when using Git and different projects, especially new projects is accidentally committing files you don't want to.
As you make changes, you may run into an issue where gitignore doesn't seem to ignore folders or files that are clearly marked to be excluded from the repository.
Below are steps to fix your repository.

.NET Core 2.2 web app in IIS
Monday, May 20, 2019
There are steps to perform before your .NET Core 2 web app can be hosted successfully through IIS.
- Install IIS if it is not already installed
- Copy your code over including the web.config file
- Create a new website within IIS if needed
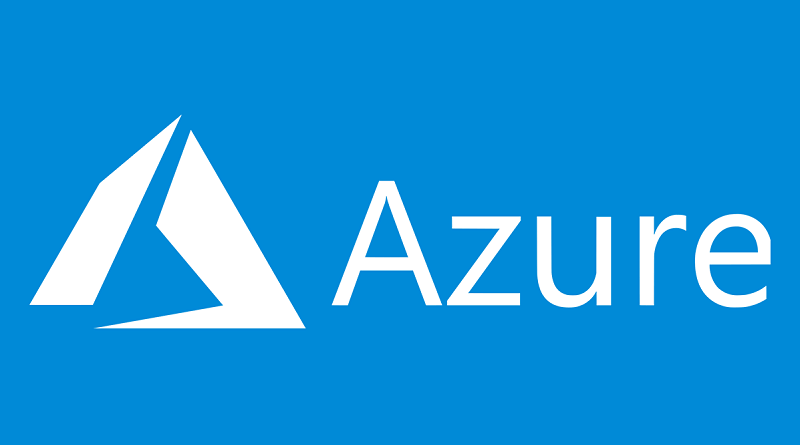
Azure Hosting - SETUP
Sunday, May 19, 2019
If you have a Visual Studio Professional subscription you are eligible to get a $50 credit each month to use for any Azure services.
To get started you'll need to create what Azure calls resources.
Create a virtual machine
Select your Subscription and your Resource group (Create New if there is none). Then select your region and the image (Windows Server 2016 Datacenter). For image size, I'm using Standard B2ms (2 vcpus, 8 GiB memory) which due to the 8 gigs of RAM makes working in RDP less painful.
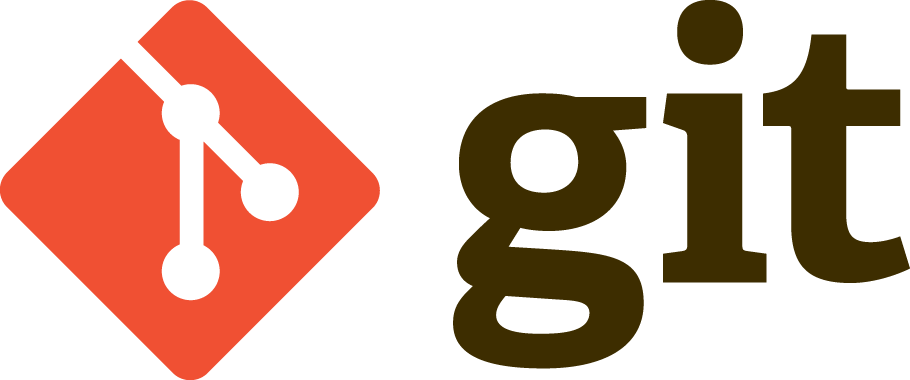
Git - Team Environment
Tuesday, May 14, 2019
Git is probably the most popular source control tool out there right now. It can be confusing at first even if you are experienced with other source code repositories (TFS, SVN, etc.) After you learn the basics and get a strong foundation, you usually realize how helpful it is and it starts to make sense.

MVC 2 Routing
Saturday, April 13, 2013
Routing
I was having problems with a URL and my controller recognizing it and found out that where you add the route within global.aspx.cs is very important.
// blog/add
routes.MapRoute(
"BlogAdd",
"blog/add",
new { controller = "BlogAdd", action = "Index", id = UrlParameter.Optional }
);
// blog
routes.MapRoute(
"Blog",
"blog/{id}",
new { controller = "Blog", action = "Index", id = "" }
);

Loading Tile Maps on Windows Phone
Friday, September 28, 2012
The problem I ran into when trying to create a Tile Mapping engine was reading data on Windows Phone. Windows Phone will not allow the below namespace so I had to go another route.
using System.Runtime.Serialization.Formatters.Binary;
Windows Phone has a class called TileContainer which allows access to the Phone's Content area.

Jquery Templates
Thursday, September 13, 2012
Using JQuery Templates to search Blogs.
Place the Jquery Templates script on the layout page or on the page using the template:
jQuery.tmpl.min.js
The JavaScript file contains the code below:

Razor Engine
Monday, August 13, 2012
My Blog model has categories associated with it (MVC3, Razor, etc.), so in order to edit which category goes with each blog I used the code below. The code below loops through each category so that they are available.
CheckCategory is a function that loops through all of the currently selected categories and returns true so that I can mark a category as being selected.
Twitter Feed - JavaScript and XML
Thursday, March 22, 2012
The LoadTweets() function pulls in a certain amount of tweets from a Twitter account. This function parses the tweet and updates links so that they are clickable.
The URL below will send an XML result. Change the publictimeline to a valid Twitter account and indicate the amount of tweets you need to receive.
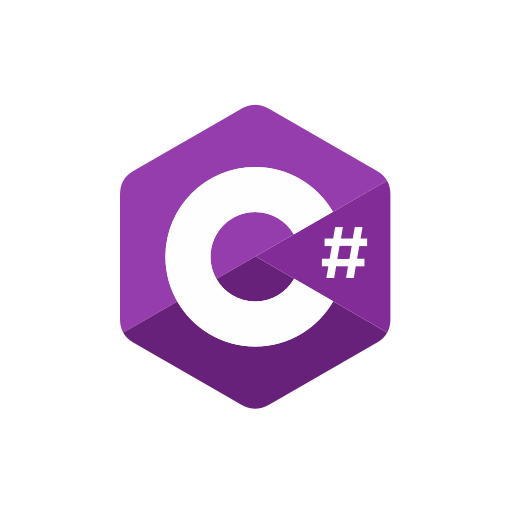
Linq to SQL LIKE
Friday, March 16, 2012
When searching and the need arises for LIKE '%query%' using Linq to SQL, use below:
[Route("search")]
[HttpPost]
public IActionResult Search(string search)
{
var posts = _db.Posts
.Where(x => x.Body.Contains(search))
.Where(x => x.Title.Contains(search)).ToArray();
ViewBag.SearchCriteria = search;
ViewBag.TagView = new TagView(_db);
return View(posts);
}
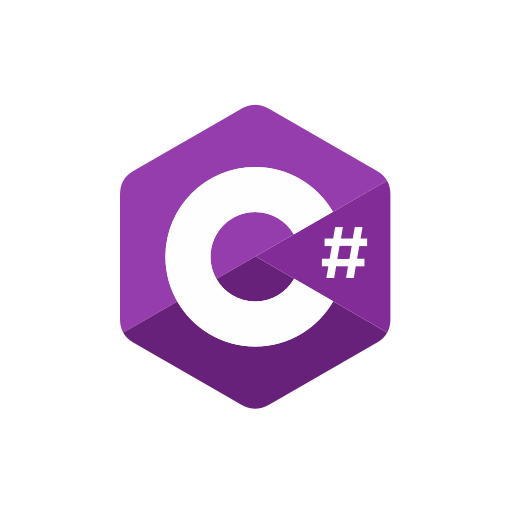
Partial Views
Tuesday, October 18, 2011
Add a View to the project. Check the "Create a partial view (.asxc)' check box, select "Create a strongly-typed view" if using a model with page and select the "View data class" if creating a strongly-type view.
%@ Control Language="C#" Inherits="System.Web.Mvc.ViewUserControl>" %>
<% foreach (var blog in Model) } %>
ASP.NET Timeout Problems
Friday, January 28, 2011
"Connection to database has timed out." This error will occur if there is a lot of processing being used by a SqlDataReader on a web page. My quick resolution was to increase the CommandTimeout on the SqlCommand object.
SqlCommand objCmd = new SqlCommand(spProcedure, objCon);
objCmd.CommandTimeout = 120;
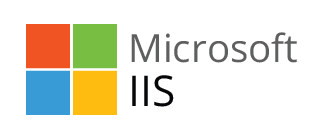
Different application pools within an application
Friday, January 28, 2011
PROBLEM:
You have an ASP.NET 4.0 website running. Within that folder structure you would like to include a separate application that is built on ASP.NET 2.0. The solution is in the web.config file.
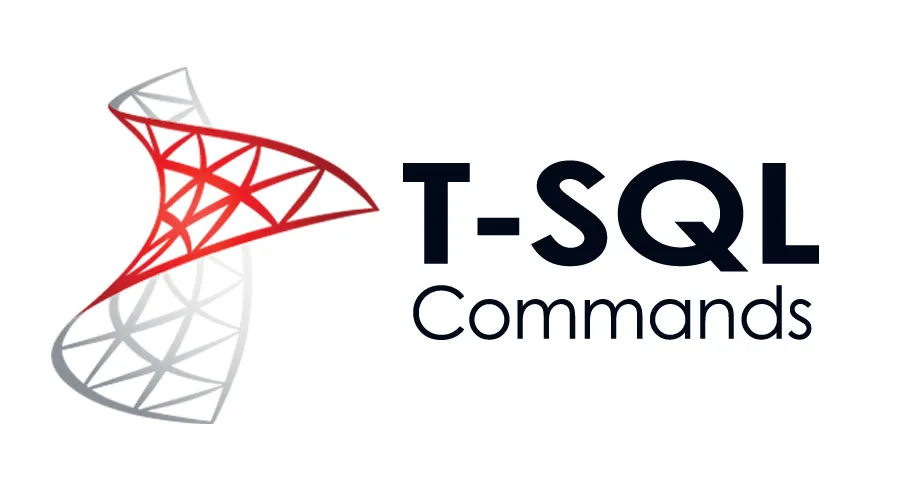
SQL Split Function
Tuesday, January 18, 2011
PROBLEM:
Had an application that contained a field in SQL Server which stored all of it's keywords as a comma delimited string. This presented a problem when doing searches by keywords and was hard to maintain. So, I wanted to move each keyword in this field into a keyword table instead of listing them all seperated by commas.
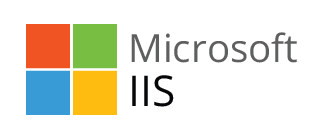
CS0016 Could not write to output file
Wednesday, December 22, 2010
Just finished creating my new ASP.NET 4 application and after I copy over the files from my development server to production, I get the dreaded "CS0016: Could not write to output file" error!
This is usually an indication that their is a permission problem of some sort. After searching for a while on google, I decided to do some of my own tests. From looking on the web, people were having success adding NETWORK SERVICE and the IIS_IUSRS accounts to the folder below:
%SYSTEMROOT%\TEMP1 = TEMP
%SYSTEMROOT%\TEMP1 = TMP

MVC Models
Tuesday, December 21, 2010
PROBLEM:
To be able to get all Blog data and Categories data into a view, both of which are stored in a database.
Popular Blogs
Docker Install and website setup
All Blogs
Blazor Server 403 Forbidden Errors
Blazor Server Timeout/Reconnect Issues
Docker Install and website setup
Loading Tile Maps on Windows Phone
Twitter Feed - JavaScript and XML
Different application pools within an application